상똥이의 Back-End 공부방
[TypeScript] 클래스 본문
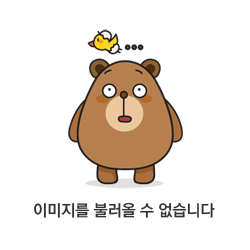
1. class 란?
- 클래스: 커스텀 자료형을 만들 수 있게 해주는 도구
(* new String(), new Array() 처럼 선언하지만, 프로그래밍 언어에 내장되지 않은 자료형을 내가 직접 만들 수 있게 해줌)
- 인스턴스: class를 new 키워드로 선언해 메모리에 저장되는 것, 커스텀한 class의 변수와 메서드를 가짐
- 변수와 메서드 선언 가능
- extends 키워드로 클래스를 상속하는 다른 클래스를 선언할 수 있음
- ES6 표준으로, 컴파일 과정을 거쳐도 유지되어 비슷한 역할을 하는 인터페이스보다 권장됨
2. 구성요소
- constructor, 접근 제한자, getter와 setter
(1) constructor
- 인스턴스별로 가지게 되는 값을 설정해주는 생성자
- 인스턴스 생성 시 constructor에 필요한 값을 파라미터로 전달
// 내가 원하는 자료형 생성
class Person {
NAME: string;
AGE: number;
ADDRESS: string;
constructor(name: string, age: number, address: string) {
this.NAME = name;
this.AGE = age;
this.ADDRESS = address;
}
}
// 자료형을 new 키워드로 가져와 인스턴스를 생성하고 메모리에 저장
// 인스턴스 생성 시 constructor에 필요한 값을 파라미터로 전달
const A = new Person("sanghee", 27, 'Seoul');
(2) 접근 제한자
- 클래스에 선언된 변수 또는 메서드의 접근 가능 범위를 설정하는 역할
- public, private, protected로 나뉨
- public: 전체 영역(클래스 내,외 및 자식 클래스)에서 접근 가능, 접근 제한자를 설정하지 않으면 public이 기본값이 됨
class Person {
NAME: string; // public 변수
constructor(name: string) {
this.NAME = name;
}
revealName() {
console.log(this.NAME); // 클래스 내부: 접근 가능
}
}
class Student extends Person {
// 자식 클래스: 접근 가능
showName() {
console.log(this.NAME);
}
}
const A = new Student('sanghee');
console.log(A.NAME); // 클래스 외부: 접근 가능
- protetected: 해당 클래스와 자식 클래스에서만 접근 가능
class Person {
protected NAME: string;
constructor(name: string) {
this.NAME = name;
}
revealName() {
console.log(this.NAME); // 클래스 내부: 접근 가능
}
}
class Student extends Person {
// 자식 클래스: 접근 가능
showName() {
console.log(this.NAME);
}
}
const A = new Student('sanghee');
console.log(A.NAME); // (오류) 클래스 외부: 접근 불가능
- private: 해당 클래스 내부에서만 접근 가능, 외부 및 자식클래스에서 접근 불가능
class Person {
private NAME: string; // private 변수
constructor(name: string) {
this.NAME = name;
}
// 클래스 내부: 접근 가능
revealName() {
console.log(this.NAME);
}
}
class Student extends Person {
// (오류) 자식 클래스: 접근 불가능
showName() {
console.log(this.NAME);
}
}
const A = new Student('sanghee');
console.log(A.NAME); // (오류) 클래스 외부: 접근 불가능
- readonly: 값 선언만 가능하며 변경이 불가능함
class Person {
readonly IS_ALIEN: boolean = false;
NAME: string;
constructor(name: string) {
this.NAME = name;
}
}
const A = new Person('sanghee');
A.IS_ALIEN = true;
- static: 전역 변수를 선언할 때 사용되며, 모든 인스턴스가 공유하는 값, 인스턴스별로 할당할 수 있는 값이 아님
class Person {
static IS_ALIEN: boolean = false;
NAME: string;
constructor(name: string) {
this.NAME = name;
}
}
const A = new Person('sanghee');
Person.IS_ALIEN = true; // 클래스 단계에서 접근 가능
const isAlien = A.IS_ALIEN; // (오류) 인스턴스에서 static 변수를 가져올 수 없음
- set : constructor의 역할과 비슷하게 인스턴스별 값을 입력해주는 것, 인자값을 하나만 받기 때문에 값을 하나씩만 설정 가능
- get : 읽기 전용 프로퍼티, private과 protect 값도 리턴 가능
class Person {
public NAME: string;
protected AGE: number;
private ADDRESS: string;
set setName(name: string) {
this.NAME = name;
}
set setAge(age: number) {
this.AGE = age;
}
set setAddress(address: string) {
this.ADDRESS = address;
}
get age() : number {
return this.AGE;
}
get address(): string {
return this.ADDRESS;
}
}
const A = new Person();
A.setName = 'sanghee';
A.setAge = 27;
A.setAddress = 'Seoul';
const A_NAME = A.getName;
const A_AGE = A.getAge;
const A_ADDRESS = A.getAddress;
** 요약
클래스 내부 | 자식 클래스 | 클래스 외부 | |
public | O | O | O |
protected | O | O | X |
private | O | X | X |
3. 상속
(1) 개념
- 클래스는 extends 키워드로 다른 클래스를 상속받아 사용할 수 있음
- 자식(하위) 클래스: 상속받은 클래스
- 부모(상위) 클래스: 상속해준 클래스
(2) super
- super()를 사용함으로써 부모 클래스의 변수를 자식 클래스에서 설정 가능
- 자식 클래스의 constructor 내부의 가장 상단에 위치해야 함
class Person {
IS_ALIEN: boolean;
NAME: string;
constructor(isAlien: boolean) {
this.IS_ALIEN = isAlien;
}
}
class Student extends Person {
SCHOOL: string;
constructor(name: string, school: string) {
super(false); // IS_ALIEN 설정
this.NAME = name;
this.SCHOOL = school;
}
}
const A = new Student('sanghee', 'Tistory School'); // NAME, SCHOOL 설정
(3) 오버라이딩
- 부모 클래스의 메서드를 자식 클래스에서 재정의하는 것
- 기능은 재정의하되, 메서드명은 동일해야 함
- 자식 클래스에 맞게 기능을 확장하고자 사용
class Person {
sayHi(): void {
console.log("Hi from parent class");
}
}
class Student extends Person {
sayHi(): string {
console.log("Hi from parent class");
return "Hi from child class";
}
}
'TypeScript' 카테고리의 다른 글
[TypeScript] 제네릭 (0) | 2024.09.03 |
---|---|
[TypeScript] 인터페이스와 타입 (0) | 2024.09.02 |
[TypeScript] 데이터 타입 (0) | 2024.09.02 |
[TypeScript] 타입스크립트 개요 (0) | 2024.09.01 |